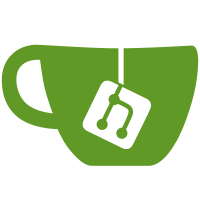
This is a major upgrade with assorted things included. - Navigation flows improved, search appears in URL history, cleared when navigating to another folder - More efficient file list format for faster loads - Efficient updates, never re-send full root another time (except at connection) - Large number of watching and filelist updates (inotify issues remain) - File size coloring - Fixed ZIP generation random glitches (thread race condition) - Code refactoring, cleanup, typing fixes - More tests Reviewed-on: #3
43 lines
747 B
TypeScript
43 lines
747 B
TypeScript
export type FUID = string
|
|
|
|
export type Document = {
|
|
loc: string
|
|
name: string
|
|
key: FUID
|
|
size: number
|
|
sizedisp: string
|
|
mtime: number
|
|
modified: string
|
|
haystack: string
|
|
dir: boolean
|
|
}
|
|
|
|
export type errorEvent = {
|
|
error: {
|
|
code: number
|
|
message: string
|
|
redirect: string
|
|
}
|
|
}
|
|
|
|
// Raw types the backend /api/watch sends us
|
|
|
|
export type FileEntry = [
|
|
number, // level
|
|
string, // name
|
|
FUID,
|
|
number, //mtime
|
|
number, // size
|
|
number, // isfile
|
|
]
|
|
|
|
export type UpdateEntry = ['k', number] | ['d', number] | ['i', Array<FileEntry>]
|
|
|
|
// Helper structure for selections
|
|
export interface SelectedItems {
|
|
keys: FUID[]
|
|
docs: Record<FUID, Document>
|
|
recursive: Array<[string, string, Document]>
|
|
missing: Set<FUID>
|
|
}
|