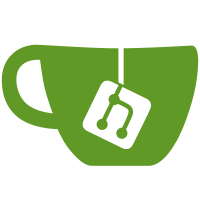
* Version * Version 20.6.1 * Fix imports and isort to remove from Makefile deprecated options * duplicate the mypy ignore hint across both lines after splitting the `from trio import ...` statement onto two lines, need to duplicate the mypy ignore hint across both lines to keep mypy from complaining Co-authored-by: Ashley Sommer <ashleysommer@gmail.com>
50 lines
1.2 KiB
Python
50 lines
1.2 KiB
Python
# Run with: gunicorn --workers=1 --worker-class=meinheld.gmeinheld.MeinheldWorker simple_server:main
|
|
""" Minimal helloworld application.
|
|
"""
|
|
import ujson
|
|
|
|
from wheezy.http import HTTPResponse, WSGIApplication
|
|
from wheezy.http.response import json_response
|
|
from wheezy.routing import url
|
|
from wheezy.web.handlers import BaseHandler
|
|
from wheezy.web.middleware import (
|
|
bootstrap_defaults,
|
|
path_routing_middleware_factory,
|
|
)
|
|
|
|
|
|
class WelcomeHandler(BaseHandler):
|
|
def get(self):
|
|
response = HTTPResponse(content_type="application/json; charset=UTF-8")
|
|
response.write(ujson.dumps({"test": True}))
|
|
return response
|
|
|
|
|
|
all_urls = [
|
|
url("", WelcomeHandler, name="default"),
|
|
# url('', welcome, name='welcome')
|
|
]
|
|
|
|
|
|
options = {}
|
|
main = WSGIApplication(
|
|
middleware=[
|
|
bootstrap_defaults(url_mapping=all_urls),
|
|
path_routing_middleware_factory,
|
|
],
|
|
options=options,
|
|
)
|
|
|
|
|
|
if __name__ == "__main__":
|
|
import sys
|
|
|
|
from wsgiref.simple_server import make_server
|
|
|
|
try:
|
|
print("Visit http://localhost:{}/".format(sys.argv[-1]))
|
|
make_server("", int(sys.argv[-1]), main).serve_forever()
|
|
except KeyboardInterrupt:
|
|
pass
|
|
print("\nThanks!")
|