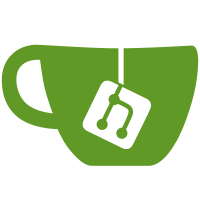
Update all tests to be compatible with requests-async Cleanup testing client changes with black and isort Remove Python 3.5 and other meta doc cleanup rename pyproject and fix pep517 error Add black config to tox.ini Cleanup tests and remove aiohttp tox.ini change for easier development commands Remove aiohttp from changelog and requirements Cleanup imports and Makefile
54 lines
1.2 KiB
Python
54 lines
1.2 KiB
Python
import asyncio
|
|
|
|
from queue import Queue
|
|
from unittest.mock import MagicMock
|
|
|
|
from sanic.response import HTTPResponse
|
|
from sanic.testing import HOST, PORT
|
|
|
|
|
|
async def stop(app, loop):
|
|
await asyncio.sleep(0.1)
|
|
app.stop()
|
|
|
|
|
|
calledq = Queue()
|
|
|
|
|
|
def set_loop(app, loop):
|
|
loop.add_signal_handler = MagicMock()
|
|
|
|
|
|
def after(app, loop):
|
|
calledq.put(loop.add_signal_handler.called)
|
|
|
|
|
|
def test_register_system_signals(app):
|
|
"""Test if sanic register system signals"""
|
|
|
|
@app.route("/hello")
|
|
async def hello_route(request):
|
|
return HTTPResponse()
|
|
|
|
app.listener("after_server_start")(stop)
|
|
app.listener("before_server_start")(set_loop)
|
|
app.listener("after_server_stop")(after)
|
|
|
|
app.run(HOST, PORT)
|
|
assert calledq.get() is True
|
|
|
|
|
|
def test_dont_register_system_signals(app):
|
|
"""Test if sanic don't register system signals"""
|
|
|
|
@app.route("/hello")
|
|
async def hello_route(request):
|
|
return HTTPResponse()
|
|
|
|
app.listener("after_server_start")(stop)
|
|
app.listener("before_server_start")(set_loop)
|
|
app.listener("after_server_stop")(after)
|
|
|
|
app.run(HOST, PORT, register_sys_signals=False)
|
|
assert calledq.get() is False
|