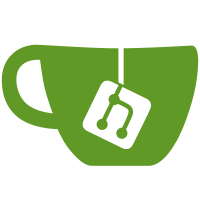
Allows for injection of the app object into the request object through the request handler. This allows for users to setup things like database connections etc. in listeners and then utilize them throughout the their various route handlers. Usage is fairly simple like so: ```python @app.get('/') async def handler(request): request.app.anything ``` Name is up in the air but I'll leave this up for a few days and I'll change it if we get a consensus
44 lines
1.1 KiB
Python
44 lines
1.1 KiB
Python
import random
|
|
|
|
from sanic import Sanic
|
|
from sanic.response import json
|
|
from ujson import loads
|
|
|
|
|
|
def test_storage():
|
|
app = Sanic('test_text')
|
|
|
|
@app.middleware('request')
|
|
def store(request):
|
|
request['user'] = 'sanic'
|
|
request['sidekick'] = 'tails'
|
|
del request['sidekick']
|
|
|
|
@app.route('/')
|
|
def handler(request):
|
|
return json({'user': request.get('user'), 'sidekick': request.get('sidekick')})
|
|
|
|
request, response = app.test_client.get('/')
|
|
|
|
response_json = loads(response.text)
|
|
assert response_json['user'] == 'sanic'
|
|
assert response_json.get('sidekick') is None
|
|
|
|
|
|
def test_app_injection():
|
|
app = Sanic('test_app_injection')
|
|
expected = random.choice(range(0, 100))
|
|
|
|
@app.listener('after_server_start')
|
|
async def inject_data(app, loop):
|
|
app.injected = expected
|
|
|
|
@app.get('/')
|
|
async def handler(request):
|
|
return json({'injected': request.app.injected})
|
|
|
|
request, response = app.test_client.get('/')
|
|
|
|
response_json = loads(response.text)
|
|
assert response_json['injected'] == expected
|