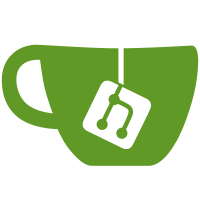
* Smarter auto fallback * remove config from blueprints * Add tests for error formatting * Add check for proper format * Fix some tests * Add some tests * docstring * Add accept matching * Add some more tests on matching * Fix contains bug, earlier return on MediaType eq * Add matching flags for wildcards * Add mathing controls to accept * Cleanup dev cruft * Add cleanup and resolve OSError relating to test implementation * Fix test * Fix some typos
63 lines
1.3 KiB
Python
63 lines
1.3 KiB
Python
from pathlib import PurePath
|
|
from typing import Dict, Iterable, List, NamedTuple, Optional, Union
|
|
|
|
from sanic.models.handler_types import (
|
|
ErrorMiddlewareType,
|
|
ListenerType,
|
|
MiddlewareType,
|
|
SignalHandler,
|
|
)
|
|
|
|
|
|
class FutureRoute(NamedTuple):
|
|
handler: str
|
|
uri: str
|
|
methods: Optional[Iterable[str]]
|
|
host: str
|
|
strict_slashes: bool
|
|
stream: bool
|
|
version: Optional[int]
|
|
name: str
|
|
ignore_body: bool
|
|
websocket: bool
|
|
subprotocols: Optional[List[str]]
|
|
unquote: bool
|
|
static: bool
|
|
version_prefix: str
|
|
error_format: Optional[str]
|
|
|
|
|
|
class FutureListener(NamedTuple):
|
|
listener: ListenerType
|
|
event: str
|
|
|
|
|
|
class FutureMiddleware(NamedTuple):
|
|
middleware: MiddlewareType
|
|
attach_to: str
|
|
|
|
|
|
class FutureException(NamedTuple):
|
|
handler: ErrorMiddlewareType
|
|
exceptions: List[BaseException]
|
|
|
|
|
|
class FutureStatic(NamedTuple):
|
|
uri: str
|
|
file_or_directory: Union[str, bytes, PurePath]
|
|
pattern: str
|
|
use_modified_since: bool
|
|
use_content_range: bool
|
|
stream_large_files: bool
|
|
name: str
|
|
host: Optional[str]
|
|
strict_slashes: Optional[bool]
|
|
content_type: Optional[bool]
|
|
resource_type: Optional[str]
|
|
|
|
|
|
class FutureSignal(NamedTuple):
|
|
handler: SignalHandler
|
|
event: str
|
|
condition: Optional[Dict[str, str]]
|