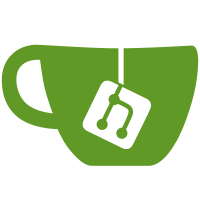
Before fix, it raises error like: ``` tests/test_utils.py F ================================= FAILURES ================================= ______________________________ test_redirect _______________________________ app = <sanic.sanic.Sanic object at 0x1045fda20>, method = 'get', uri = '/1', gather_request = True, debug = False server_kwargs = {}, request_args = (), request_kwargs = {} _collect_request = <function sanic_endpoint_test.<locals>._collect_request at 0x1045ec950> _collect_response = <function sanic_endpoint_test.<locals>._collect_response at 0x1045ec7b8> def sanic_endpoint_test(app, method='get', uri='/', gather_request=True, debug=False, server_kwargs={}, *request_args, **request_kwargs): results = [] exceptions = [] if gather_request: def _collect_request(request): results.append(request) app.request_middleware.appendleft(_collect_request) async def _collect_response(sanic, loop): try: response = await local_request(method, uri, *request_args, **request_kwargs) results.append(response) except Exception as e: exceptions.append(e) app.stop() app.run(host=HOST, debug=debug, port=PORT, after_start=_collect_response, **server_kwargs) if exceptions: raise ValueError("Exception during request: {}".format(exceptions)) if gather_request: try: > request, response = results E ValueError: too many values to unpack (expected 2) sanic/utils.py:47: ValueError During handling of the above exception, another exception occurred: utils_app = <sanic.sanic.Sanic object at 0x1045fda20> def test_redirect(utils_app): """Test sanic_endpoint_test is working for redirection""" > request, response = sanic_endpoint_test(utils_app, uri='/1') tests/test_utils.py:33: _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ app = <sanic.sanic.Sanic object at 0x1045fda20>, method = 'get', uri = '/1', gather_request = True, debug = False server_kwargs = {}, request_args = (), request_kwargs = {} _collect_request = <function sanic_endpoint_test.<locals>._collect_request at 0x1045ec950> _collect_response = <function sanic_endpoint_test.<locals>._collect_response at 0x1045ec7b8> def sanic_endpoint_test(app, method='get', uri='/', gather_request=True, debug=False, server_kwargs={}, *request_args, **request_kwargs): results = [] exceptions = [] if gather_request: def _collect_request(request): results.append(request) app.request_middleware.appendleft(_collect_request) async def _collect_response(sanic, loop): try: response = await local_request(method, uri, *request_args, **request_kwargs) results.append(response) except Exception as e: exceptions.append(e) app.stop() app.run(host=HOST, debug=debug, port=PORT, after_start=_collect_response, **server_kwargs) if exceptions: raise ValueError("Exception during request: {}".format(exceptions)) if gather_request: try: request, response = results return request, response except: raise ValueError( "Request and response object expected, got ({})".format( > results)) E ValueError: Request and response object expected, got ([{}, {}, {}, <ClientResponse(http://127.0.0.1:42101/3) [200 OK]> E <CIMultiDictProxy('Content-Type': 'text/plain; charset=utf-8', 'Content-Length': '2', 'Connection': 'keep-alive', 'Keep-Alive': 'timeout=1')> E ]) sanic/utils.py:52: ValueError ```
97 lines
2.5 KiB
Python
97 lines
2.5 KiB
Python
import pytest
|
|
|
|
from sanic import Sanic
|
|
from sanic.response import text, redirect
|
|
from sanic.utils import sanic_endpoint_test
|
|
|
|
|
|
@pytest.fixture
|
|
def redirect_app():
|
|
app = Sanic('test_redirection')
|
|
|
|
@app.route('/redirect_init')
|
|
async def redirect_init(request):
|
|
return redirect("/redirect_target")
|
|
|
|
@app.route('/redirect_init_with_301')
|
|
async def redirect_init_with_301(request):
|
|
return redirect("/redirect_target", status=301)
|
|
|
|
@app.route('/redirect_target')
|
|
async def redirect_target(request):
|
|
return text('OK')
|
|
|
|
@app.route('/1')
|
|
def handler(request):
|
|
return redirect('/2')
|
|
|
|
@app.route('/2')
|
|
def handler(request):
|
|
return redirect('/3')
|
|
|
|
@app.route('/3')
|
|
def handler(request):
|
|
return text('OK')
|
|
|
|
return app
|
|
|
|
|
|
def test_redirect_default_302(redirect_app):
|
|
"""
|
|
We expect a 302 default status code and the headers to be set.
|
|
"""
|
|
request, response = sanic_endpoint_test(
|
|
redirect_app, method="get",
|
|
uri="/redirect_init",
|
|
allow_redirects=False)
|
|
|
|
assert response.status == 302
|
|
assert response.headers["Location"] == "/redirect_target"
|
|
assert response.headers["Content-Type"] == 'text/html; charset=utf-8'
|
|
|
|
|
|
def test_redirect_headers_none(redirect_app):
|
|
request, response = sanic_endpoint_test(
|
|
redirect_app, method="get",
|
|
uri="/redirect_init",
|
|
headers=None,
|
|
allow_redirects=False)
|
|
|
|
assert response.status == 302
|
|
assert response.headers["Location"] == "/redirect_target"
|
|
|
|
|
|
def test_redirect_with_301(redirect_app):
|
|
"""
|
|
Test redirection with a different status code.
|
|
"""
|
|
request, response = sanic_endpoint_test(
|
|
redirect_app, method="get",
|
|
uri="/redirect_init_with_301",
|
|
allow_redirects=False)
|
|
|
|
assert response.status == 301
|
|
assert response.headers["Location"] == "/redirect_target"
|
|
|
|
|
|
def test_get_then_redirect_follow_redirect(redirect_app):
|
|
"""
|
|
With `allow_redirects` we expect a 200.
|
|
"""
|
|
response = sanic_endpoint_test(
|
|
redirect_app, method="get",
|
|
uri="/redirect_init", gather_request=False,
|
|
allow_redirects=True)
|
|
|
|
assert response.status == 200
|
|
assert response.text == 'OK'
|
|
|
|
|
|
def test_chained_redirect(redirect_app):
|
|
"""Test sanic_endpoint_test is working for redirection"""
|
|
request, response = sanic_endpoint_test(redirect_app, uri='/1')
|
|
assert request.url.endswith('/1')
|
|
assert response.status == 200
|
|
assert response.text == 'OK'
|
|
assert response.url.endswith('/3')
|