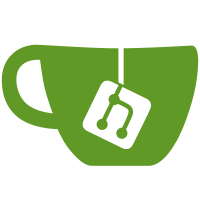
* HTTP1 header formatting moved to headers.format_headers and rewritten. - New implementation is one line of code and twice faster than the old one. - Whole header block encoded to UTF-8 in one pass. - No longer supports custom encode method on header values. - Cookie objects now have __str__ in addition to encode, to work with this. * Linter * format_http1_response * Replace encode_body with faster implementation based on f-string. Benchmarks: def encode_body(data): try: # Try to encode it regularly return data.encode() except AttributeError: # Convert it to a str if you can't return str(data).encode() def encode_body2(data): return f"{data}".encode() def encode_body3(data): return str(data).encode() data_str, data_int = "foo", 123 %timeit encode_body(data_int) 928 ns ± 2.96 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each) %timeit encode_body2(data_int) 280 ns ± 2.09 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each) %timeit encode_body3(data_int) 387 ns ± 1.7 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each) %timeit encode_body(data_str) 202 ns ± 1.9 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each) %timeit encode_body2(data_str) 197 ns ± 0.507 ns per loop (mean ± std. dev. of 7 runs, 10000000 loops each) %timeit encode_body3(data_str) 313 ns ± 1.28 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each) * Wtf linter * Content-type fixes. * Body encoding sanitation, first pass. - body/data type autodetection fixed. - do not repr(body).encode() bytes-ish values. - support __html__ and _repr_html_ in sanic.response.html(). * <any type>-to-str response autoconversion limited to sanic.response.text() only. * Workaround MyPy issue. * Add an empty line to make isort happy. * Add html test for __html__ and _repr_html_. * Remove StreamingHTTPResponse.get_headers helper function. * Add back HTTPResponse Keep-Alive removed by earlier merge or something. * Revert "Remove StreamingHTTPResponse.get_headers helper function." Tests depend on this otherwise useless function. This reverts commit 9651e6ae017b61bed6dd88af6631cdd6b01eb347. * Add deprecation warnings; instead of assert for wrong HTTP version, and for non-string response.text. * Add back missing import. * Avoid duplicate response header tweaking code. * Linter errors
.. image:: https://raw.githubusercontent.com/huge-success/sanic-assets/master/png/sanic-framework-logo-400x97.png :alt: Sanic | Build fast. Run fast. Sanic | Build fast. Run fast. ============================= .. start-badges .. list-table:: :stub-columns: 1 * - Build - | |Build Status| |AppVeyor Build Status| |Codecov| * - Docs - |Documentation| * - Package - | |PyPI| |PyPI version| |Wheel| |Supported implementations| |Code style black| * - Support - | |Forums| |Join the chat at https://gitter.im/sanic-python/Lobby| |Awesome| * - Stats - | |Downloads| |Conda downloads| .. |Forums| image:: https://img.shields.io/badge/forums-community-ff0068.svg :target: https://community.sanicframework.org/ .. |Join the chat at https://gitter.im/sanic-python/Lobby| image:: https://badges.gitter.im/sanic-python/Lobby.svg :target: https://gitter.im/sanic-python/Lobby?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge .. |Codecov| image:: https://codecov.io/gh/huge-success/sanic/branch/master/graph/badge.svg :target: https://codecov.io/gh/huge-success/sanic .. |Build Status| image:: https://travis-ci.org/huge-success/sanic.svg?branch=master :target: https://travis-ci.org/huge-success/sanic .. |AppVeyor Build Status| image:: https://ci.appveyor.com/api/projects/status/d8pt3ids0ynexi8c/branch/master?svg=true :target: https://ci.appveyor.com/project/huge-success/sanic .. |Documentation| image:: https://readthedocs.org/projects/sanic/badge/?version=latest :target: http://sanic.readthedocs.io/en/latest/?badge=latest .. |PyPI| image:: https://img.shields.io/pypi/v/sanic.svg :target: https://pypi.python.org/pypi/sanic/ .. |PyPI version| image:: https://img.shields.io/pypi/pyversions/sanic.svg :target: https://pypi.python.org/pypi/sanic/ .. |Code style black| image:: https://img.shields.io/badge/code%20style-black-000000.svg :target: https://github.com/ambv/black .. |Wheel| image:: https://img.shields.io/pypi/wheel/sanic.svg :alt: PyPI Wheel :target: https://pypi.python.org/pypi/sanic .. |Supported implementations| image:: https://img.shields.io/pypi/implementation/sanic.svg :alt: Supported implementations :target: https://pypi.python.org/pypi/sanic .. |Awesome| image:: https://cdn.rawgit.com/sindresorhus/awesome/d7305f38d29fed78fa85652e3a63e154dd8e8829/media/badge.svg :alt: Awesome Sanic List :target: https://github.com/mekicha/awesome-sanic .. |Downloads| image:: https://pepy.tech/badge/sanic/month :alt: Downloads :target: https://pepy.tech/project/sanic .. |Conda downloads| image:: https://img.shields.io/conda/dn/conda-forge/sanic.svg :alt: Downloads :target: https://anaconda.org/conda-forge/sanic .. end-badges Sanic is a **Python 3.6+** web server and web framework that's written to go fast. It allows the usage of the ``async/await`` syntax added in Python 3.5, which makes your code non-blocking and speedy. `Source code on GitHub <https://github.com/huge-success/sanic/>`_ | `Help and discussion board <https://community.sanicframework.org/>`_. The project is maintained by the community, for the community. **Contributions are welcome!** The goal of the project is to provide a simple way to get up and running a highly performant HTTP server that is easy to build, to expand, and ultimately to scale. Installation ------------ ``pip3 install sanic`` Sanic makes use of ``uvloop`` and ``ujson`` to help with performance. If you do not want to use those packages, simply add an environmental variable ``SANIC_NO_UVLOOP=true`` or ``SANIC_NO_UJSON=true`` at install time. .. code:: shell $ export SANIC_NO_UVLOOP=true $ export SANIC_NO_UJSON=true $ pip3 install --no-binary :all: sanic .. note:: If you are running on a clean install of Fedora 28 or above, please make sure you have the ``redhat-rpm-config`` package installed in case if you want to use ``sanic`` with ``ujson`` dependency. Hello World Example ------------------- .. code:: python from sanic import Sanic from sanic.response import json app = Sanic() @app.route('/') async def test(request): return json({'hello': 'world'}) if __name__ == '__main__': app.run(host='0.0.0.0', port=8000) Sanic can now be easily run using ``python3 hello.py``. .. code:: [2018-12-30 11:37:41 +0200] [13564] [INFO] Goin' Fast @ http://0.0.0.0:8000 [2018-12-30 11:37:41 +0200] [13564] [INFO] Starting worker [13564] And, we can verify it is working: ``curl localhost:8000 -i`` .. code:: HTTP/1.1 200 OK Connection: keep-alive Keep-Alive: 5 Content-Length: 17 Content-Type: application/json {"hello":"world"} **Now, let's go build something fast!** Documentation ------------- `Documentation on Readthedocs <http://sanic.readthedocs.io/>`_. Changelog --------- `Release Changelogs <https://github.com/huge-success/sanic/blob/master/CHANGELOG.rst>`_. Questions and Discussion ------------------------ `Ask a question or join the conversation <https://community.sanicframework.org/>`_. Contribution ------------ We are always happy to have new contributions. We have `marked issues good for anyone looking to get started <https://github.com/huge-success/sanic/issues?q=is%3Aopen+is%3Aissue+label%3Abeginner>`_, and welcome `questions on the forums <https://community.sanicframework.org/>`_. Please take a look at our `Contribution guidelines <https://sanic.readthedocs.io/en/latest/sanic/contributing.html>`_.
Description
Languages
Python
66.1%
CSS
19.5%
Sass
7.4%
JavaScript
6.3%
SCSS
0.5%