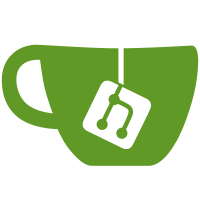
* Socket binding implemented properly for IPv6 and UNIX sockets. - app.run("::1") for IPv6 - app.run("unix:/tmp/server.sock") for UNIX sockets - app.run("localhost") retains old functionality (randomly either IPv4 or IPv6) Do note that IPv6 and UNIX sockets are not fully supported by other Sanic facilities. In particular, request.server_name and request.server_port are currently unreliable. * Fix Windows compatibility by not referring to socket.AF_UNIX unless needed. * Compatibility fix. * Fix test of existing unix socket. * Cleaner unix socket removal. * Remove unix socket on exit also with workers=1. * More pedantic UNIX socket implementation. * Refactor app to take unix= argument instead of unix:-prefixed host. Goin' fast @ unix-socket fixed. * Linter * Proxy properties cleanup. Slight changes of semantics. SERVER_NAME now overrides everything. * Have server fill in connection info instead of request asking the socket. - Would be a good idea to remove request.transport entirely but I didn't dare to touch it yet. * Linter 💣🌟✊💀 * Fix typing issues. request.server_name returns empty string if host header is missing. * Fix tests * Tests were failing, fix connection info. * Linter nazi says you need that empty line. * Rename a to addr, leave client empty for unix sockets. * Add --unix support when sanic is run as module. * Remove remove_route, deprecated in 19.6. * Improved unix socket binding. * More robust creating and unlinking of sockets. Show proper and not temporary name in conn_info. * Add comprehensive tests for unix socket mode. * Hide some imports inside functions to avoid Windows failure. * Mention unix socket mode in deployment docs. * Fix merge commit. * Make test_unix_connection_multiple_workers pickleable for spawn mode multiprocessing. Co-authored-by: L. Kärkkäinen <tronic@users.noreply.github.com> Co-authored-by: Adam Hopkins <admhpkns@gmail.com>
74 lines
2.2 KiB
Python
74 lines
2.2 KiB
Python
import os
|
|
import sys
|
|
|
|
from argparse import ArgumentParser
|
|
from importlib import import_module
|
|
from typing import Any, Dict, Optional
|
|
|
|
from sanic.app import Sanic
|
|
from sanic.log import logger
|
|
|
|
|
|
def main():
|
|
parser = ArgumentParser(prog="sanic")
|
|
parser.add_argument("--host", dest="host", type=str, default="127.0.0.1")
|
|
parser.add_argument("--port", dest="port", type=int, default=8000)
|
|
parser.add_argument("--unix", dest="unix", type=str, default="")
|
|
parser.add_argument(
|
|
"--cert", dest="cert", type=str, help="location of certificate for SSL"
|
|
)
|
|
parser.add_argument(
|
|
"--key", dest="key", type=str, help="location of keyfile for SSL."
|
|
)
|
|
parser.add_argument("--workers", dest="workers", type=int, default=1)
|
|
parser.add_argument("--debug", dest="debug", action="store_true")
|
|
parser.add_argument("module")
|
|
args = parser.parse_args()
|
|
|
|
try:
|
|
module_path = os.path.abspath(os.getcwd())
|
|
if module_path not in sys.path:
|
|
sys.path.append(module_path)
|
|
|
|
module_parts = args.module.split(".")
|
|
module_name = ".".join(module_parts[:-1])
|
|
app_name = module_parts[-1]
|
|
|
|
module = import_module(module_name)
|
|
app = getattr(module, app_name, None)
|
|
app_name = type(app).__name__
|
|
|
|
if not isinstance(app, Sanic):
|
|
raise ValueError(
|
|
f"Module is not a Sanic app, it is a {app_name}. "
|
|
f"Perhaps you meant {args.module}.app?"
|
|
)
|
|
if args.cert is not None or args.key is not None:
|
|
ssl = {
|
|
"cert": args.cert,
|
|
"key": args.key,
|
|
} # type: Optional[Dict[str, Any]]
|
|
else:
|
|
ssl = None
|
|
|
|
app.run(
|
|
host=args.host,
|
|
port=args.port,
|
|
unix=args.unix,
|
|
workers=args.workers,
|
|
debug=args.debug,
|
|
ssl=ssl,
|
|
)
|
|
except ImportError as e:
|
|
logger.error(
|
|
f"No module named {e.name} found.\n"
|
|
f" Example File: project/sanic_server.py -> app\n"
|
|
f" Example Module: project.sanic_server.app"
|
|
)
|
|
except ValueError:
|
|
logger.exception("Failed to run app")
|
|
|
|
|
|
if __name__ == "__main__":
|
|
main()
|