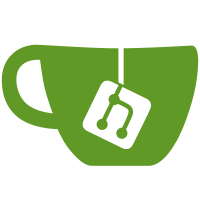
The Streaming section of the docs was updated to make clear that a synchronous write should be used in the callback, but this section was not updated.
1.6 KiB
1.6 KiB
Response
Use functions in sanic.response
module to create responses.
Plain Text
from sanic import response
@app.route('/text')
def handle_request(request):
return response.text('Hello world!')
HTML
from sanic import response
@app.route('/html')
def handle_request(request):
return response.html('<p>Hello world!</p>')
JSON
from sanic import response
@app.route('/json')
def handle_request(request):
return response.json({'message': 'Hello world!'})
File
from sanic import response
@app.route('/file')
async def handle_request(request):
return await response.file('/srv/www/whatever.png')
Streaming
from sanic import response
@app.route("/streaming")
async def index(request):
async def streaming_fn(response):
response.write('foo')
response.write('bar')
return response.stream(streaming_fn, content_type='text/plain')
Redirect
from sanic import response
@app.route('/redirect')
def handle_request(request):
return response.redirect('/json')
Raw
Response without encoding the body
from sanic import response
@app.route('/raw')
def handle_request(request):
return response.raw('raw data')
Modify headers or status
To modify headers or status code, pass the headers
or status
argument to those functions:
from sanic import response
@app.route('/json')
def handle_request(request):
return response.json(
{'message': 'Hello world!'},
headers={'X-Served-By': 'sanic'},
status=200
)